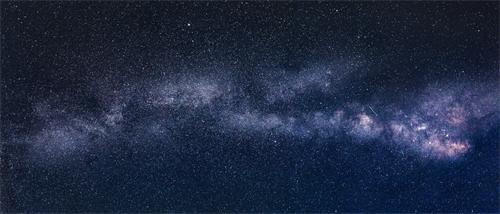
The complete guide to WebSockets with React
Full duplex means that data can be sent either way on the connection at any time. WebSocket is a modern way to have persistent browser-server connections. You can also download it (upper-right button in the iframe) and run it locally. Just don’t forget to install Node.js and npm install ws before running. But the data will be buffered (stored) in memory and sent out only as fast as network speed allows.
Apidog is a simple and powerful tool that allows you to test and debug WebSocket connections. If you’re using the web side and need to debug local services, you’ll need to install the Google plugin for Apidog. WebSocket with JavaScript is very easy to use with JavaScript, the most popular scripting language for the web. JavaScript has a built-in object called WebSocket that provides a simple and intuitive API to create and manage WebSocket connections. WebSockets are one of the most interesting and convenient ways to achieve real-time capabilities in a modern application. They give us a lot of flexibility to leverage full-duplex communications.
Why to use websocket and what is the advantage of using it?
Start proactively monitoring your React Native apps — try LogRocket for free. Here the link corresponds to the socket service running on the backend. This article aims to present the advantages and benefits WebSocket brings to the table, as well as its disadvantages and limitations. That’s all for enabling WSS from the programming perspective, but from the networking perspective, you have to generate cryptographic keys and certificates via a trusted Certificate Authority (CA). For more information on perfect-cursors, you can check out the official documentation over on GitHub.
- WebSockets are similar to SSE but also triumph in taking messages back from the client to the server.
- Or, an attacker could use a WebSocket to launch a denial-of-service attack by flooding the server with requests.
- Both the client and the web server can initiate the closing handshake.
- As a final touch, let’s leverage the list of users to render a crude “who’s online list”.
By monitoring and logging WebSocket traffic, you can quickly detect when something unusual is happening and take appropriate action. The server is terminating the connection due to a temporary condition, e.g., it is overloaded. Serves as a heartbeat mechanism ensuring the connection is still alive. Every frame has an opcode that determines how to interpret that frame’s payload data. The standard opcodes currently in use are defined by RFC 6455 and maintained by the Internet Assigned Numbers Authority (IANA). The WebSocket protocol makes fragmentation possible via the first bit of the WebSocket frame — the FIN bit, which indicates whether the frame is the final fragment in a message.
How to use WebSockets with React and Node (WebSocket tutorial)
You can then use this information to decide whether or not you need to take action to reduce memory usage. When you have a chatty protocol, it means that there is a lot of small data being sent back and forth between the client and server. This can quickly add up to a lot of data being transferred, which can cause performance issues.
At the top, we’ll create a horizontal bar to show connection or disconnection notifications as well as errors. At the bottom, we’ll place an input field and a submit button to send messages through WebSockets. The rest of the middle area will be used to display the list of messages received from the server. In a request-response policy, the client looks for a response in the sent request. This means the client knows when it will get data from the server so it stays ready to handle it.
Implementing the WebSocket server in Node
As you can see, setting up the WebSocket connection with useWebSocket is easy enough. If you recall, the server has been coded to extract the username query parameter to identify the user. In the following video I quickly recap how the server works by sending and subscribing to data using an API platform Postman. Additionally, we initialize an empty state object which we will later populate with information about the user’s status or attributes.
For example, Slack uses WebSockets for instant messaging between chat users. You can think of a WebSocket connection as a long-lived, bidirectional, full-duplex communication channel between a web client and a web server. On the other hand, shipping production-ready realtime functionality powered by open-source WebSocket what is websocket libraries is not at easy as building a simple demo app. See, for example, the many engineering challenges involved in scaling Socket.IO, one of the most popular open-source WebSocket libraries out there. The server was acting as a gateway or proxy and received an invalid response from the upstream server.
Top 10 Backend Frameworks for 2024
Ably offers versatile, easy-to-use APIs to develop powerful realtime apps. In this post, we built a fullstack JavaScript WebSocket application that works great on localhost or with a small set of users. Rather than jump straight into the tutorial, we started with a focus on the fundamentals. This way, no matter what specific shape your application takes, you can feel confident about the best way to manage the WebSocket connection in React.
However, in many cases, applications need to communicate across different networks. For example, an application running on a phone might need to communicate with an application running on a server in a different country. The endpoint is terminating the connection because it received a message that violates its policy.
Connection restrictions are no longer an issue because data is served over a single TCP socket connection. The very first attempt to solve the problem was by polling the server at regular intervals. The normal polling approach fetches data from the server frequently based on an interval defined on the client side (typically using setInterval or recursive setTimeout). On the other hand, the long polling approach is similar to normal polling, but the server handles the timeout/waiting time. The WebSocket protocol offers persistent, real-time, full-duplex communication between the client and the server over a single TCP socket connection.
We will walk you through how to implement WebSockets in React Native by designing a message broadcast app. For example, if a chat app needs login/signup, make sure to let only authenticated users establish WebSocket connections by validating a token before the HTTP handshake succeeds. At the client level, I use the React useWebSocket library to initiate a WebSocket connection.
When I was learning about WebSockets in React, this caused me a bit of anxiety! I went looking for a definitive best practice but, as it happens, there isn’t a universal “right” answer. It depends on what you’re building and the specific shape of your application. They are a great choice when two-way communication is needed such as chat and multiplayer collaboration. It supports falling back to HTTP long-polling if the connection is lost and will try to reconnect automatically. WebSockets provide a full-duplex connection that stays alive until the server/client drops the connection.